Ambient intelligence(Aml) is explained by wikipedia as
In computing, ambient intelligence (AmI) refers to electronic environments that are sensitive and responsive to the presence of people.In an ambient intelligence world, devices work in concert to support people in carrying out their everyday life activities, tasks and rituals in easy, natural way using information and intelligence that is hidden in the network connecting these
devices.
Its also called pervasive computing or ubiquitous computing.We can build up Necromancer-like future visions of ultra sci-fi devices roaming invisibly around us mumbling 0s and 1s wrapping us inside its matrix... In the Wikipedia , you can read an interesting
scenario about the use of ambient intelligence.This idea will be used by most gadgets now in market.. all those smart home appliances,wifi devices, mobiles,RFID, GPS etc.There is a lot to know about the concepts and implementation (complex middle-ware architectures ) for Aml.
In the web world some recent works brought everyone's attention are location aware technology standards and APIs.Geographical Information systems was here for a longtime.Those tools like ARCGIS are prominent in the field.After the Gmap revolution hit the web, there was such an insurge of developing applications based on the geo spatial information.The virtual representation of real world is always the cyber punk readers dreams.The recent technological innovations do make them viable .
Yes the web browsers are the ubiquitous application which will connect the real space to virtual one.Now most mobile devices have browsers.So the web is getting ready for the opera of next generation location aware applications.W3C has now recently released the draft of
Geolocation API specification standard.
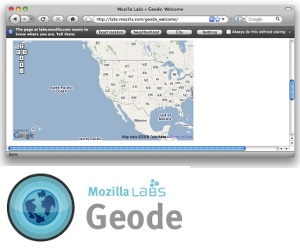
Mozilla has developed
Geode,an experimental add-on to explore geo-location in Firefox 3. It includes a single experimental geolocation service provider so that any computer with WiFi can get accurate positioning data.
Yahoo! Fire Eagle is a service that acts as a broker for your location, creating a single place where any web service, from any device can get access to your last updated location.service enables you to share your geographic position over many different applications, websites and services. You can update your location with your GPS-enabled phone (e.g. iPhone 3G) or any other software that integrates with FireEagle and you can allow websites and services to use this information.
Google released its
Geo location API for google Gears .The Geolocation API provides the best

estimate of the user's position using a number of sources (called location providers). These providers may be on board(GPS for example) or server-based (a network location provider).
So it is possible to use these apis to develop environmentally intelligent applications in devices especially mobile .It is possible to read my feeds / news based on locality ... like reading The Times of India epaper from Bangalore edition or Hyderabad edition based on location while I am traveling.
What about a web based Advertising platform based on location ..?
No wonder why Google urge to allocate ambient spaces for wifi ...
free the airwaves !! These technologies will give numerous possibilities for Android development
Cartography did give the light to Renaissance.It did make revolutions,wars,civilizations ... Now the neo-geographers is leading the world to the cyberspace navigation.World is again shrinking... an invisible revolution ?
The ambient intelligence is realized by the human centric products than technology centric products.The information technology is now spreading from big corporates dealing with the mammoth analytical data and transactions to common man...where the data is unimaginably galactic.. geo-spatial data.. the paradigm of consumerism enabled by the new generation web ideologies. These ubiquitous location aware devices could form the new era of invisible computing
a term invented by
Donald Norman in his book
The Invisible Computer published a decade ago to describe the coming age of ubiquitous task-specific computing devices. The devices are so highly optimized to particular tasks that they blend into the world and require little technical knowledge on the part of their users.

image from
here